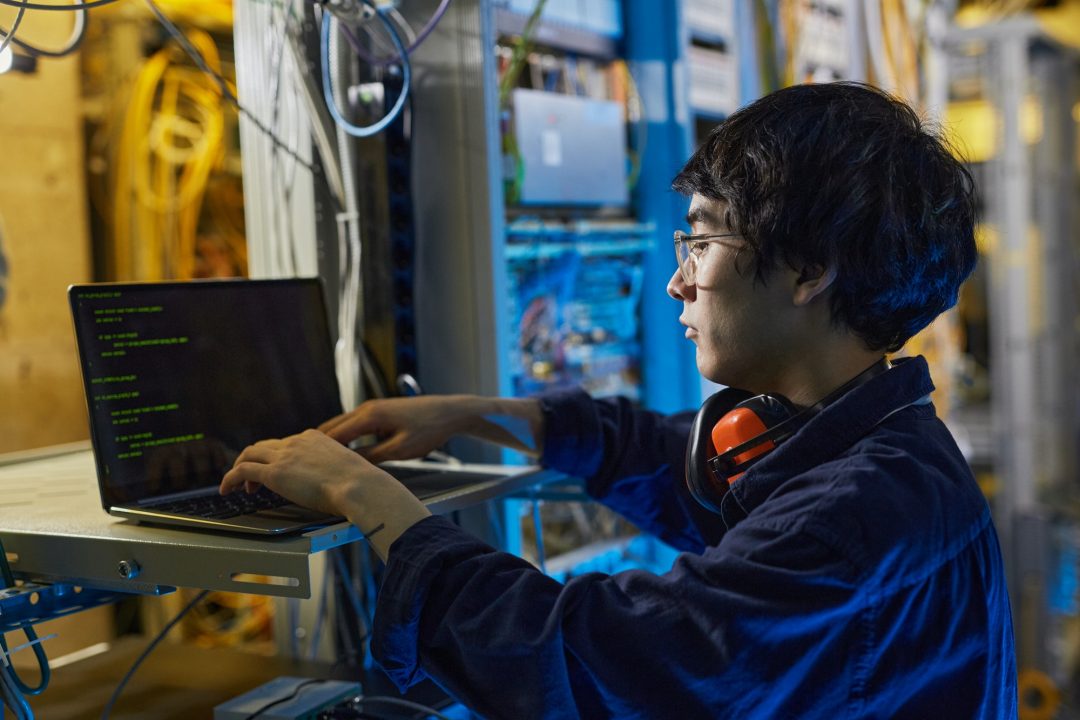
Introduction
Welcome to the gateway of excellence in engineering education at BloomBerg EduBerg! At our renowned institution, we pave the path for aspiring engineers to soar to new heights of success.
Embark on a journey of discovery and innovation as we delve into the core disciplines of engineering. From Computer Science and Engineering, Artificial Intelligence, and Data Science to Information Technology, our comprehensive curriculum is tailored to meet the demands of the rapidly evolving tech landscape.
At BloomBerg EduBerg, our tuition for core engineering courses transcends traditional boundaries, blending academic rigor with hands-on experience and real-world applications. Our distinguished faculty members, industry experts, and state-of-the-art facilities provide the perfect ecosystem for learning and growth.
Join us as we redefine the future of engineering education. Discover your passion, unleash your potential, and become a catalyst for change in the ever-evolving world of engineering. Your journey to greatness starts here, at BloomBerg EduBerg – where innovation knows no bounds.
Programming in C
(Common to all disciplines)
- Module 1 : Application Software & System software: Compilers, interpreters, High level and low level languages, Introduction to structured approach to programming, Flow chart Algorithms, Pseudo code (bubble sort, linear search - algorithms and pseudocode)
- Module 2 : Basic structure of C program: Character set, Tokens, Identifiers in C, Variables and Data Types, Constants, Console IO Operations, printf and scanf. Operators and Expressions: Expressions and Arithmetic Operators, Relational and Logical Operators, Conditional operator, size of operator, Assignment operators and Bitwise Operators. Operators Precedence Control Flow Statements: If Statement, Switch Statement, Unconditional Branching using goto statement, While Loop, Do While Loop, For Loop, Break and Continue statements (Simple programs covering control flow)
- Module 3 : Arrays Declaration and Initialization, 1-Dimensional Array, 2-Dimensional Array. String processing: In built String handling functions (strlen, strcpy, strcat and strcmp, puts, gets) Linear search program, bubble sort program, simple programs covering arrays and strings.
- Module 4 : Introduction to modular programming, writing functions, formal parameters, actual parameters Pass by Value, Recursion, Arrays as Function Parameters structure, union, Storage Classes, Scope and life time of variables, simple programs using functions
- Module 5 :Basics of Pointer: declaring pointers, accessing data though pointers, NULL pointer, array access using pointers, pass by reference effect. File Operations: open, close, read, write, append. Sequential access and random access to files: In built file handling functions (rewind(), fseek(), ftell(), feof(), fread(), fwrite()), simple programs covering pointers and files.
Programming in Java
- Module 1 : Introduction to Java programming – Classes fundamentals, objects, methods, constructors, parameter passing, overloading, access control keywords– static members -Comments, Arrays- Java Documentation usage
- Module 2 : Inheritance – Super classes- sub classes –Protected members – constructors in sub classesthe Object class – abstract classes and methods- final methods and classes – Interfaces – defining an interface, implementing interface, differences between classes and interfaces and extending interfaces – Defining and importing packages. Strings-Java Built-in Classes and it's usage
- Module 3 : Exceptions – exception hierarchy – throwing and catching exceptions – built-in exceptions, creating own exceptions, Stack Trace Elements. Input / Output Basics – Streams – Byte streams and Character streams – Reading and Writing Console – Reading and Writing Files
- Module 4 : Differences between multithreading and multitasking, thread life cycle, creating threads, synchronizing threads, Inter-thread communication. Generic Programming – Generic classes – generic methods – Bounded Types – Restrictions and Limitations
- Module 5 : Graphics programming – Frame – Components – working with 2D shapes – Using color, fonts, and images – Basics of event handling – event handlers – adapter classes – actions – mouse events – AWT event hierarchy – Introduction to Swing – layout management – Swing Components – Text Fields , Text Areas – Buttons- Check Boxes – Radio Buttons – Listschoices- Scrollbars – Windows –Menus – Dialog Boxes.
Programming in Python
- Module 1 : Introduction To Python: Understanding Python-identifiers, variables, keywords, expressions and statements, evaluation of expressions, Operators and operands, operator precedence, indentation. Python Program Flow Control: Decision making- if, if..else, elif. Loops - for, while, for...else, while...else, Control statements using pass, continue, break.
- Module 2 : Strings and lists – string traversal, string slices and comparison with examples, The string module, character classification. List- List values, accessing elements, list membership, Lists and for loops, List operations, List slices, List deletion, Matrices. Tuples - mutability and tuples, tuple assignment, Tuples as return values, Tuple operations. Dictionaries – operations and methods.
- Module 3 : Python Functions, Modules and Packages: Function definition, calling functions, parameters and arguments, the return statement, type conversion and coercion, composition of functions, Lambda function, mathematical functions, user-defined functions, Recursion, Modules- Built- in modules, creating modules, import statement. Packages in Python - importing modules from a package.
- Module 4 : Python Files and exceptions: Python file handling, open, write, read text files, writing variables, Directories in Python, Pickling, Exception Handling.
- Module 5 : Python Object Oriented Programming: Introduction to classes and objects – class definition, attributes, instances, sameness, instances as arguments and return values. Constructor, class attributes and destructors, Inheritance.