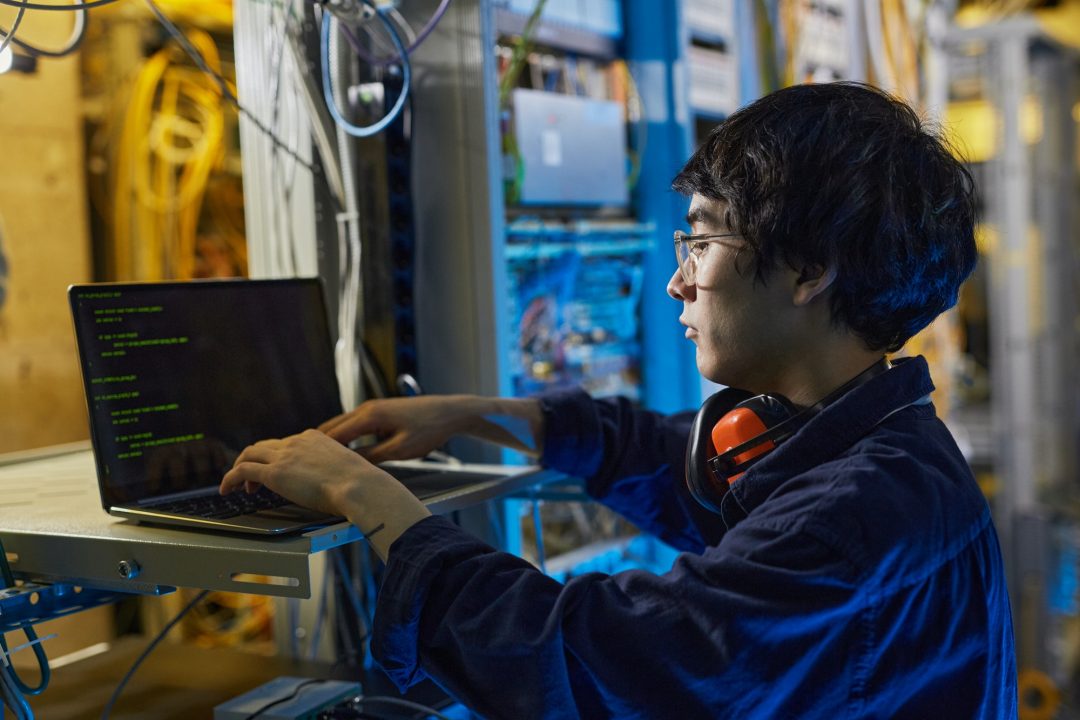
Introduction
The Full Stack Python Web Development course is designed to equip learners with the skills needed to build dynamic, data-driven web applications using Python as the backend language. This course covers both frontend and backend development, enabling students to become proficient in full-stack development. With practical projects and hands-on exercises, learners will gain the skills to work with web frameworks, databases, authentication, APIs, and deployment techniques to create end-to-end web applications.
Course Objectives
Master frontend development with HTML, CSS, JavaScript.
Learn backend development using Python with frameworks like Flask and Django.
Work with databases (SQL and NoSQL) and integrate them into web applications.
Implement user authentication and authorization systems.
Develop full-stack applications with best practices in coding and design.
Syllabus Outline
Module 1: Introduction to Web Development
- Overview of Full Stack Development.
- Introduction to frontend and backend technologies.
- Understanding HTTP and how web browsers communicate with servers.
- Introduction to Python for web development.
- Setting up development environments: VS Code, Git, GitHub, and virtual environments.
Module 2: Frontend Development Basics
- HTML: Structure of web pages (elements, tags, attributes).
- CSS: Styling web pages (layout, positioning, typography).
- JavaScript: Client-side scripting (DOM manipulation, event handling).
Module 3: Backend Development with Python
- Introduction to Django web framework.
- Django: Setting up Django projects, Models, Views, and Templates (MVT).
- Working with URLs, routing, and templates.
- Introduction to Python’s database connectors (SQLite, MySQL, PostgreSQL).
Module 4: Databases for Web Development
- Introduction to SQL
- Working with SQLite, MySQL, and PostgreSQL in Django.
- Database Modelling and relationships (one-to-many, many-to-many).
- Introduction to ORM (Object-Relational Mapping) using SQLAlchemy and Django ORM.
- CRUD operations (Create, Read, Update, Delete).
Module 5: User Authentication and Authorization
- Implementing User Registration and Login functionality.
- Handling sessions and cookies.
- Introduction to JWT (JSON Web Tokens) for secure authentication.
- Implementing user authorization and roles (admin, user, etc.).
- OAuth integration for third-party login (Google, Facebook).
Module 6: APIs and RESTful Services
- Introduction to APIs (Application Programming Interfaces).
- Building RESTful APIs with Django.
- Handling HTTP Methods: GET, POST, PUT, DELETE.
- API Authentication with JWT and OAuth.
- Introduction to GraphQL: Building queries and mutations.
- Using Postman for testing APIs.
Module 7: Frontend Integration with Backend
- Integrating Frontend (HTML, CSS, JavaScript) with Django backend.
- Sending HTTP requests from the frontend to the backend using Axios or Fetch API.
- Handling responses and updating the UI dynamically based on data from the backend.
- Managing state in the frontend using JavaScript (for dynamic content rendering).
- Building interactive web pages by connecting frontend with backend APIs for data-driven content.
Module 8: Project Development and Best Practices
- Building a full-stack web application: Combining everything learned in the course.
- Version control with Git and GitHub for project management.
- Testing web applications: Unit testing and integration testing.
- Best practices in code structure, debugging, and documentation.
- Agile development principles for real-world projects.
Learning Outcomes
By the end of this course, learners will be able to:
- Build dynamic and scalable full-stack web applications using Python.
- Work with modern backend framework like Django.
- Manage databases with SQL.
- Implement secure authentication and authorization systems.
- Develop and consume RESTful and GraphQL APIs.
- Deploy full-stack applications to the cloud and follow best practices for development and deployment.
Course Duration
24 weeks (depending on full-time or part-time schedule)